Calculation Object Examples
This section will show fully featured examples of each object type with the resulting calculation report and design portal view.
Examples Of Each object
ComparisonForced
Warning
This has been renamed to ComparisonStatement. The api is largely the same, but for details see https://youandvern.github.io/efficalc/base_classes.html#efficalc.ComparisonStatement
Code Example:

Calculation Report:
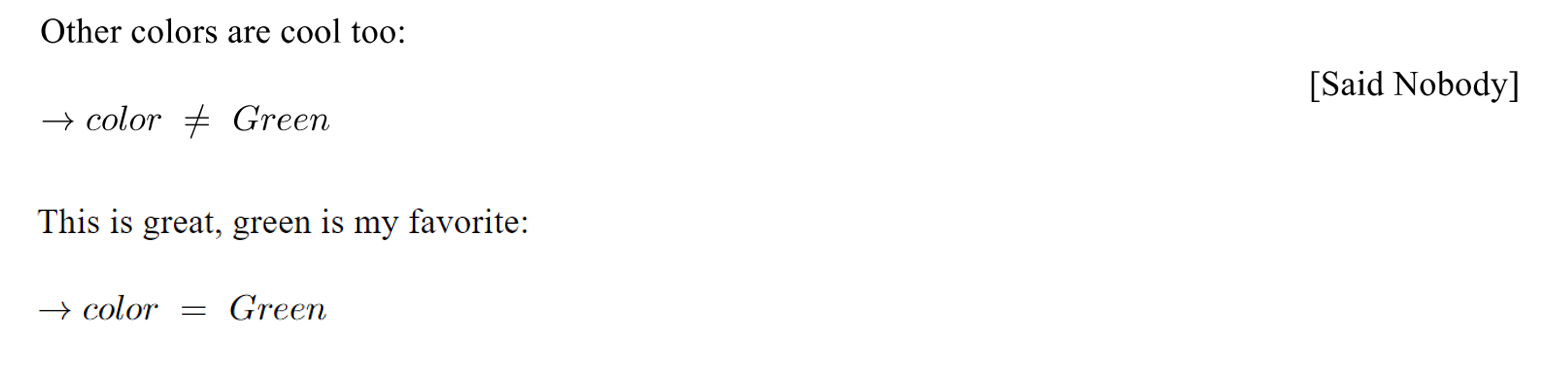
Design Portal: Not Displayed
Full Example
Warning
Some aspects of this example may be using deprecated class and function names. For up-to-date examples, view the efficalc library examples: https://github.com/youandvern/efficalc/tree/main/examples
Code
1from templates.encomp_utils import *
2
3
4def calculation():
5
6 Title("Example Calculation Title")
7
8 TextBlock(text="This can be a description of the calculation, and introduction to the author, or any other text.", reference="Author")
9
10
11 Heading(text="Inputs", head_level=4, numbered=False, reference="")
12
13 s1 = Input('l_1', 4, 'in', "The length of one side of the rectangle", min_value=0.001, max_value=100)
14
15 a = Input(variable_name="A", default_value=16, unit="in^2", description="Area of a real life small rectangle", reference="AIHM 17.3.5",
16 input_type="number", select_options=None, min_value=0, max_value=100, num_step=1)
17
18 c = Input("color", "Blue", "", "Color of the rectangle", input_type="select", select_options=["Red", "Green", "Blue", "Purple"])
19
20
21 Heading("Assumptions", 4, False)
22
23 Assumption("The rectangle in question is 2-dimensional planar")
24 Assumption("Both side lengths are greater than 0")
25 Assumption("This is a third important assumption")
26
27
28 Heading("Calculations")
29
30 TextBlock("Text blocks can add text anywhere you might need it.")
31
32 Heading("Important Calculations", 2)
33
34 s2 = Calculation("l_2", a / s1, "in", "The length of the other side of the rectangle")
35
36 h = Calculation("h", SQRT(s1**2 + s2**2), "in", "The length of the hypotenuse (rectangle diagonal)", "Pythagoras ~500BC", True)
37
38 Heading("Other Calculations", 2)
39 a_s = Calculation(variable_name="A_{square}", expression=s1**2, unit="in^2", description="The area of a small imaginary square",
40 reference="", result_check=True)
41
42
43 Heading("Design Checks")
44 Comparison(a=s1, comparator="=", b=s2, true_message="Square", false_message="Non-square", description="What type of rectangle is it?",
45 reference="", result_check=False)
46
47 Comparison(h, ">", s2, description="The hypotenuse should always be larger than the side", result_check=True)
48 Comparison(a, "<", a_s, description="I hope the square with side 1 is bigger than te rectangle", result_check=True)
49
50 if c.get_value() == "Green":
51 ComparisonForced(a=c, comparator="=", b="Green", comparator2=None, c=None, description="This is great, green is my favorite")
52 else:
53 ComparisonForced(c, "!=", "Green", description="Other colors are cool too", reference="Said Nobody")
54
55 Heading("Placeholder for future section")
56 Heading("A Sub-section", 2)
57 Heading("A sub-sub-section", 3)
58 Heading("Another sub-sub-section", 3)
59 Heading("Another sub-section", 2)
Report
This is the complete Calculation Report for the above example.
Design Portal
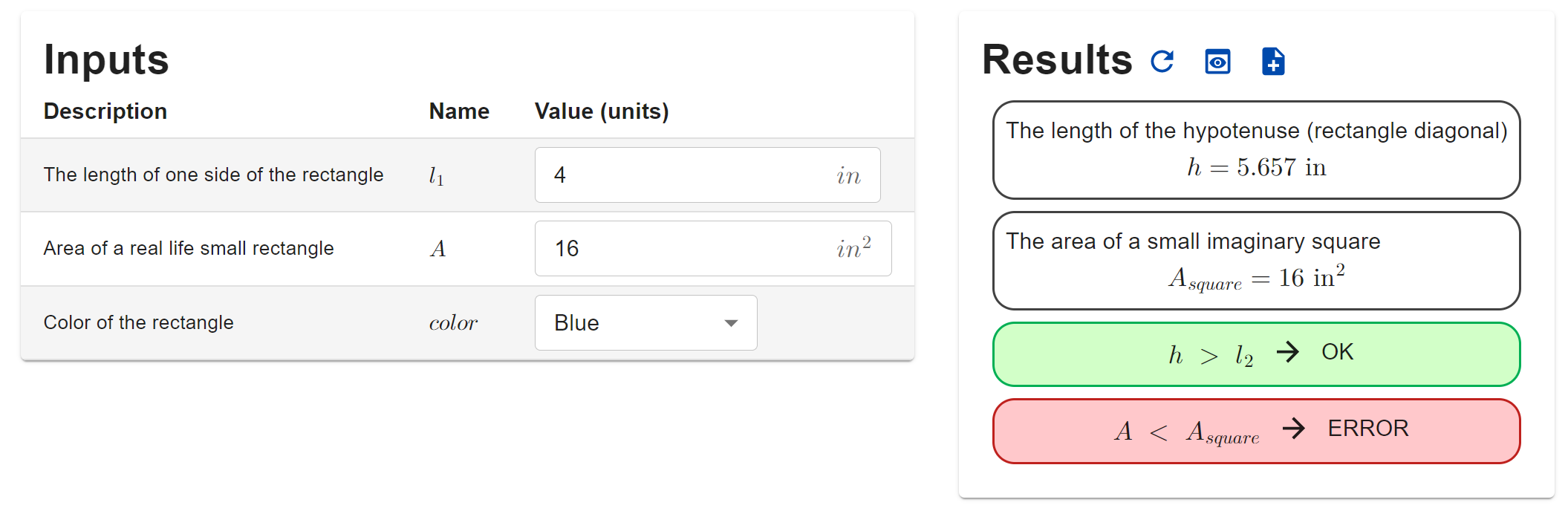